Implementing Data Structures: A Detailed Exploration
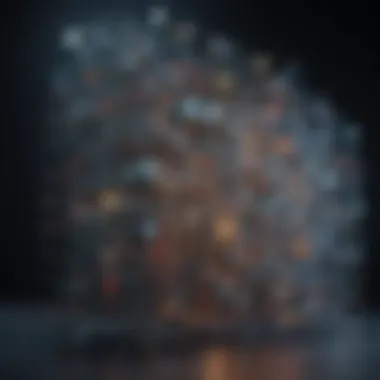
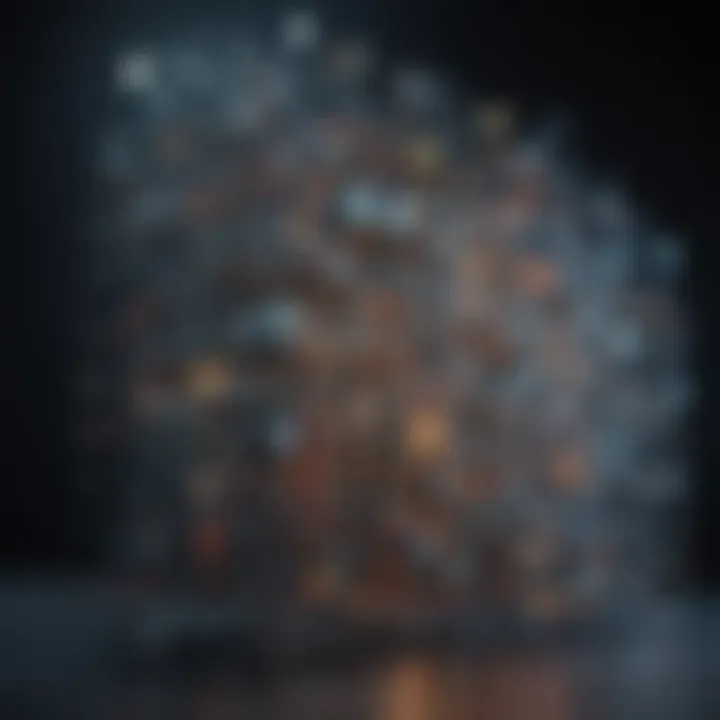
Research Overview
When we look at the world of computer science, the importance of data structures cannot be overstated. They are the backbone of how data is organized, accessed, and manipulated. Understanding data structures is akin to learning the rules of a language before you can have a meaningful conversation. This section aims to synthesize key findings related to their implementation and application, giving you, the reader, a clear understanding of why this topic is essential.
Summary of Key Findings
Data structures come in various formats, ranging from simple arrays to complex linked lists and trees. Each type has its advantages and disadvantages depending on the context of use. Some key findings include:
- Performance Efficiency: Certain data structures lead to faster performance for specific tasks. For instance, hash tables can provide average-case constant-time complexity for lookups.
- Memory Usage: Different structures use memory more efficiently than others. For instance, a sparse matrix representation can save memory when dealing with large matrices with few non-zero elements.
- Complex Problem-Solving: Advanced data structures, like graphs, play a vital role in complex problem-solving scenarios such as network routing and social network analysis.
Importance of the Research
The significance of exploring data structures extends beyond cognitive understanding. In practical terms, choosing the right data structure can mean the difference between a program that runs smoothly and one that crashes or performs poorly. Students and professionals alike benefit from grasping these concepts to craft more efficient and effective code. By digging into data structures, we uncover essential knowledge for optimization across diverse fields, from academia to industry.
"The choice of data structure typically affects the efficiency of an algorithm, sometimes to a greater extent than the actual algorithm itself."
Methodology
This article doesn't just offer insights; it also illustrates how these data structures can be systematically analyzed and implemented within various realms including academic research, software engineering, and real-world applications.
Study Design
The design for studying data structures focuses on two main axes: theoretical foundations and practical applications. While theoretical foundations introduce different data structures and their properties, practical applications assess their effectiveness in real-world situations. Students will learn not only how to choose the correct structure but also how to implement it effectively.
Data Collection Techniques
To compile insights and gathering empirical evidence:
- Literature Review: An extensive review of existing literature on data structures, algorithms, and their combined efficiencies was undertaken.
- Case Studies: Practical examples and case studies from various fields were explored to understand common challenges and solutions.
- Surveys and Interviews: Engaging with practitioners and researchers to gather firsthand accounts of their experiences and challenges related to data structures has added depth and context to the findings.
In closing, this overview sets the stage for you to understand the profound impact of data structures whether you're coding, conducting research, or developing software solutions.
Prologue to Data Structures
Data structures are the backbone of any computational task, acting as the framework that dictates how data is organized, accessed, and manipulated. When diving into this subject, one must understand that data structures are not just mere constructs; they represent a realm where efficiency meets complexity. In this section, we'll explore the essential elements of data structures, detailing why they are pivotal in the broader scope of computer science.
Defining Data Structures
At its core, a data structure is a way of storing and organizing data in a computer so it can be accessed and modified efficiently. Think of data structures like different kinds of containers, each fit for a specific kind of job. For instance, an array might be likened to a row of boxes, where each box can hold a piece of data and can be easily accessed by its index. On the other hand, a linked list resembles a chain of nodes, where each node points to the next, allowing for dynamic memory allocation.
There are various types of data structuresâsome linear, like arrays and linked lists, others non-linear, such as trees and graphs. The choice of which data structure to use often hinges on the specific requirements of the application at hand. For example, if you're working with a dataset thatâs frequently accessed and doesnât change often, you might lean towards arrays for their quick access times. But, if you need a structure that can grow and shrink as data is added or removed, linked lists are a better bet.
Importance in Computer Science
The significance of data structures in computer science cannot be overstated. They serve as the foundation upon which algorithms operate and thus are crucial for optimizing both performance and resource management. Using the right data structure can drastically improve speed and efficiency, thereby saving valuable computing resources.
"A well-chosen data structure can mean the difference between a program that runs in seconds and one that runs in hours."
For instance, consider a scenario where a software application needs to frequently search for information. Choosing a hash table can improve search times significantly compared to using a simple array. Similarly, for hierarchical datasets, a tree structure enables easier navigation than a flat list. Such optimizations are particularly essential in high-demand fields like gaming, databases, and artificial intelligence, where performance can determine the difference between success and failure.
In summary, diving into the world of data structures may feel overwhelming at first, but its importance shines through as it fosters a deeper understanding of how data operates within the computer realm. Their implications stretch far beyond academic boundaries, influencing real-world applications and advancing technology in remarkable ways.
Types of Data Structures
Understanding the various types of data structures is critical in the field of computer science. The implementation of data structures influences the performance of algorithms and dictates how data can be accessed and manipulated. Choosing the appropriate data structure can enhance efficiency, reduce complexity, and address specific application needs effectively. Different types of data structures, including linear and non-linear structures, offer unique strengths and weaknesses that must be weighed based on the task at hand.
Linear Data Structures
Linear data structures represent a straightforward arrangement of data elements. Every element is connected to its previous and next element, forming a clear sequence. This characteristic simplifies many operations, making them easier to implement but limiting the structureâs ability to handle complex relationships.
Arrays
Arrays are considered one of the most fundamental data structures used in programming. They consist of a collection of items, all of the same type, arranged in a contiguous block of memory. The key characteristic of arrays is their fixed size; once defined, their length cannot be altered. This is both an advantage and a disadvantage.
On one hand, arrays offer rapid access to their elements, thanks to their predictable memory allocation. For example, accessing the third element in an array is a constant time operation, O(1), which is highly efficient.
However, a unique feature of arrays is their rigidity concerning size. If a program requires more items than an initial array can hold, a new, larger array must be allocated, and the old items need to be copied over. This process can be cumbersome, especially in performance-critical applications.
Linked Lists
Unlike arrays, linked lists allow for flexible storage and easier data manipulation. A linked list is composed of nodes, each containing data and a reference (or pointer) to the next node in the sequence. The key characteristic of linked lists is their dynamic size, allowing for efficient memory use since nodes can be added or removed without shifting others.
In terms of unique features, linked lists allow for more memory-efficient insertions and deletions since these operations donât necessitate a complete reallocation of memory. However, this flexibility comes with disadvantages; specifically, accessing elements in a linked list is slower, with a linear time complexity of O(n), as it may require traversal through the list.
Stacks
Stacks are another linear data structure that operates on a Last In First Out (LIFO) principle. Think of a stack like a stack of plates: the last plate placed on top is the first one to be removed. The key characteristic here is that stack operations mainly consist of push (adding an item) and pop (removing an item).
Stacks are particularly beneficial for certain algorithms, such as backtracking algorithms and parsing expressions. Their unique feature is how they manage function calls in programming languagesâtracking active functions and returning from them appropriately. The downside, however, comes in the form of limited access; you often can only access the top element directly, making it less versatile than some other structures.
Queues
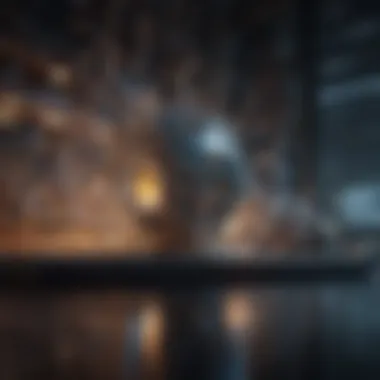
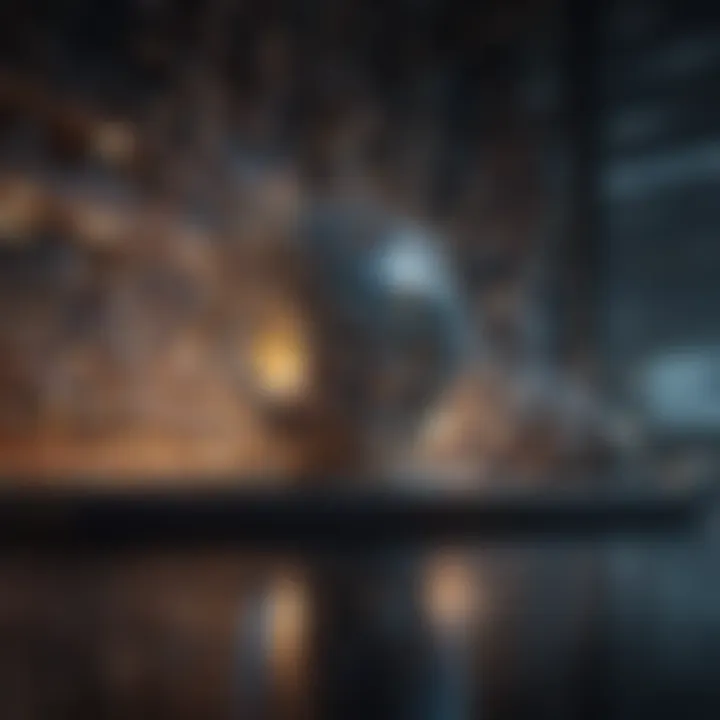
Queues are yet another form of linear data structure, following a First In First Out (FIFO) approach. It works just like a line at a coffee shop: the first person to get in line is the first to be served. The key characteristic of queues is their orderly management of data, allowing for straightforward processing affairs, making them suitable for scenarios like task scheduling and bandwidth management.
The unique feature of queues is their simple enqueue (adding an item) and dequeue (removing an item) process. However, they also have downsidesâmaintaining a dynamic size can incur overhead, and like stacks, accessing arbitrary elements is not straightforward.
Non-Linear Data Structures
Non-linear data structures, in contrast, allow for various arrangements of data elements that enable complex relationships. This flexibility is essential for applications like social networks or hierarchical data representation. Whether itâs trees or graphs, the key to understanding these structures lies in how they organize data to navigate diverse relationships.
Trees
Trees are one of the most widely used non-linear structures. They consist of nodes connected by edges, forming a hierarchy. The key characteristic of trees is that they start with a root node and branches out to child nodes. This characteristic makes them extraordinarily good at representing hierarchical relationships, like folders and files on a computer.
One notable aspect of trees is their capacity for efficient search and management operations. For example, binary search trees allow for quick searches, insertions, and deletions with average time complexities of O(log n). However, trees can become imbalanced over time, leading to potential inefficiencies in those operations.
Graphs
Graphs extend the concept of trees into more complex interconnections. A graph consists of nodes, also known as vertices, connected by edges, allowing for cyclical paths and diverse relationships. The key characteristic of graphs is their ability to represent networks, making them ideal for modeling relationships in social networks or transportation routes.
Their unique feature comes from the many algorithms available, like Dijkstra's algorithm for the shortest path finding, highlighting their usefulness in real-world applications. However, this complexity can also be a drawback. Implementing graphs can demand substantial memory, especially if storing them as adjacency matrices.
Hash Tables
Finally, hash tables provide an exceptional way to manage large data sets through a key-value pair system. The key characteristic of hash tables is their ability to provide quick access to items using a hash function, which transforms keys into indexes. This characteristic ensures average time complexities of O(1) for search, insert, and delete operations, making hash tables extremely efficient.
The unique feature of hash tables is their reliance on handling collisions, which can occur when different keys generate the same index. While several methods, such as chaining or open addressing, can manage collisions, they may affect performance. Efficient management of hash tables is critical and can become a disadvantage if not implemented correctly.
Fundamental Operations
In the realm of data structures, fundamental operations serve as the backbone of interactive functionality. These operations not only enable effective data management but also enhance the performance and efficiency of applications, providing a solid foundation for advanced algorithms. Understanding these operations is crucial because they dictate how data moves, updates, and is accessed, which can ultimately impact the overall effectiveness of a software solution.
Insertion and Deletion
Insertion and deletion are two sides of the same coin when it comes to managing data in any data structure. The process of inserting involves adding new elements, whereas deletion focuses on removing existing ones. Both operations are fundamental, yet they present unique challenges and considerations.
In the context of linked lists, for example, an insertion operation may simply require re-pointing pointers, making it a relatively efficient procedure. However, when it comes to arrays, inserting a new element may necessitate shifting existing elements to maintain order, which can be time-consuming, especially in larger datasets.
Similarly, deletion varies with the data structure; removing an element in a tree might require rebalancing, while in a queue, itâs just removing the front element. The efficiency of these operations directly affects the scalability and speed of applications, thus emphasizing their importance in fundamental operations.
Searching Algorithms
Linear Search
A linear search, as straightforward as it sounds, scans through each element of a list sequentially until the target element is found or the list is exhausted. Its most significant trait is simplicity. This method doesnât require any prior sorting of the array, making it suitable for unsorted data sets.
Although stagnant in speedâexhibiting a time complexity of O(n)âthe linear search keeps its charm in easy implementation and low overhead. The trade-off lies in its inefficiency for large datasets, where its performance diminishes noticeably. Yet, in terms of reliability, this search remains a go-to choice when dealing with smaller, unsorted collections.
Binary Search
Binary search, contrastingly, thrives on the principle of divide and conquer, requiring a sorted dataset. It halves the dataset with each iteration, focusing on the middle element to determine which half to search next. This technique elevates performance, reaching a time complexity of O(log n), which makes it a strikingly more efficient choice for large, sorted arrays.
Yet, the necessity for pre-sorting can be both a boon and a bane. While it significantly reduces search time, sorting itself can introduce additional overhead, especially for smaller datasets. The unique feature here is its ability to quickly skip half of the elements, converting lengthy search processes into swifter resolutions, although it does come at the cost of requiring a sorted array to work effectively.
Traversal Techniques
Traversal techniques are essential for exploring data structures, allowing for systematic processing of elements. They provide insight into how data can be accessed and manipulated effectively.
Breadth-First Search
Breadth-first search (BFS), with its methodical layer-by-layer exploration, is particularly valuable in scenarios where the shortest path is desired, such as in GPS navigation systems. It utilizes a queue to keep track of nodes, progressively expanding to each adjacent node before moving deeper. This iterative approach ensures that all nodes at the current depth are explored before advancing, allowing for comprehensive data assessment.
The BFS excels in finding the shortest path in unweighted graphs. However, the need for additional memory to maintain the queue can be a disadvantage, particularly with large datasets, where performance may falter.
Depth-First Search
On the flip side, the depth-first search (DFS) dives deep into one branch of data structures before backtracking. Utilizing a stack, either explicitly or through recursion, it permits a more exhaustive exploration of potential pathways. This technique shines in locating solutions in complex mazes or puzzles, where it ventures far on one path before reevaluating and trying alternatives.
Though its approach can lead to longer search times in vast structuresâpotentially culminating in running out of memory in extreme casesâit maintains a lightweight memory footprint. The unique feature here is its ability to handle rightly branching data efficiently, making it appropriate for applications requiring thorough exploration without the overhead of breadth-first methods.
In summary, mastering these fundamental operations is imperative for anyone diving into data structures, as they lay the groundwork for effectively implementing and manipulating data.
Implementation Strategies
When it comes to implementing data structures, having a solid strategy is crucial for effective software development. This section emphasizes important considerations for selecting and using data structures in programming. An appropriate implementation strategy ensures that the structure aligns with the specific needs of a project and optimizes performance, reliability, and maintenance.
Choosing the Right Structure
Selecting the correct data structure is fundamental. Various factors must be considered, such as the type of data to be stored, how it will be accessed, and the operations that will be performed. Here are some key points to think about:
- Performance Needs: Some operations may require quicker access times, making it essential to analyze which data structure can provide that efficiency. For example, a hash table allows for average case constant time complexity for lookups, making it suitable for applications requiring fast retrieval.
- Memory Usage: It's not just about speed; memory consumption plays a significant role. Some structures, like linked lists, may use more memory due to pointers, while arrays might be more efficient in space.
- Data Relationships: The inherent relationships between data items should also guide your choice. Trees can represent hierarchical relationships, and graphs represent networking connections comprehensively.
"Choosing the right data structure is the backbone of effective programming. Itâs where optimization begins."
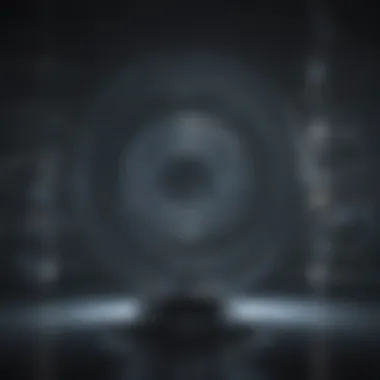
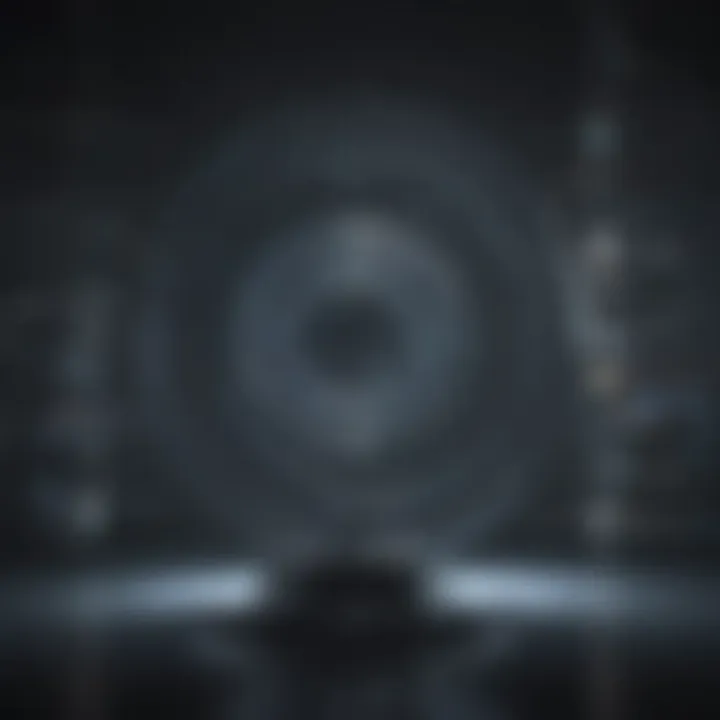
Programming Languages and Performance
Programming language choice can significantly impact how well data structures function in practice. Each language brings unique capabilities and limitations.
++
C++ is widely regarded for its fine-grained control over system resources. One of the major characteristics of C++ is its ability to perform low-level memory manipulation, which can be advantageous when implementing complex data structures. The unique feature of C++ is its performance optimization capabilities, which come from powerful features like pointers and templates.
However, while C++ offers speed and efficiency, it comes with a steep learning curve. Managing memory manually means programmers must be diligent to avoid leaks or other issues. Still, mastering this complexity often leads to highly efficient implementations.
Python
Python stands out for its simplicity and ease of use. One key characteristic is its dynamic typing, which allows developers to write less code while maintaining clarity. Python provides built-in data structures like lists and dictionaries, making it a beneficial choice for rapid development.
However, this convenience may come at the cost of performance. Python is often slower than some compiled languages like C++, especially for large-scale data manipulations. In scenarios where performance is critical, Python's elegance can sometimes be a trade-off.
Java
Java, with its âwrite once, run anywhereâ philosophy, emphasizes portability. A significant characteristic of Java is its built-in garbage collection and automatic memory management, offering simplicity in ensuring memory safety. This helps developers to focus more on algorithms rather than worrying about memory allocation.
Nonetheless, Java can sometimes lead to performance overhead due to the additional abstraction layers. While the garbage collector relieves some burdens, it may unexpectedly affect timing-sensitive applications.
In summary, the choice of programming language has a profound effect on the implementation of data structures. Each option brings its own strengths and potential downsides, so selecting the right tool for the job is critical.
Performance Analysis
Assessing the performance of data structures is a cornerstone in understanding their efficiency in various applications. It's not merely about what's good on paper; the practical implications of how data structures handle operations can make or break the efficiency of an algorithm in real-world scenarios. Factors like time complexity and space complexity are vital metrics. They inform decisions not just on the selection of data structures but also on algorithm design and optimization strategies.
Time Complexity
In computational terms, time complexity measures the time an algorithm takes to complete based on the input size. It encapsulates how an algorithm's runtime scales as data increases, helping programmers estimate performance effectively.
Big O Notation
Big O notation serves as a universal language for describing the upper bound of an algorithm's runtime as the input grows. This makes it a popular choice for performance analysis, as its expression allows coders to ignore constants and lesser-order terms, focusing only on the most impactful portion of the runtime.
What's integral to Big O is its characteristic as a worst-case scenario operator. It's not merely beneficialâit's practically essential for evaluations. This is mainly because it provides a clearer understanding of performance under load. For example:
- O(n): linear time, where performance grows linearly with data size.
- O(n^2): quadratic time, where performance deteriorates significantly as input size increases.
A unique feature of Big O notation is its ability to categorize algorithms into classes like constant, logarithmic, linear, and exponential time complexities, each offering insights into how quickly they can process data. However, one must also consider its limitations. While itâs great for high-level analysis, it doesn't offer specific runtime guarantees, often leaving developers with a gap in practical applications.
"Understanding Big O notation is a key part of algorithm efficiencyâit's the lens through which we measure potential performance hits and gains."
Averaging Case Analysis
On the flip side, averaging case analysis provides a broader perspective by considering the expected performance of an algorithm across multiple scenarios. This helps in grasping how an algorithm performs not just in the best and worst outcomes, but in the average situation.
The distinctive trait of averaging case analysis is its ability to yield insights into behavior during typical usage, making it beneficial for applications where predictable performance is crucial. For instance, when running a search algorithm on a dataset, many implementations will show better-than-worst-case complexity on average.
Letâs break down its implications in performance analysis:
- Key characteristic: It typically uses probabilistic models to account for various input distributions.
- Benefits: It helps in more realistic performance expectations, especially in system designs that anticipate varied data sets.
- Drawbacks: While it's rich in theory, it often involves complex calculations that may not always translate smoothly, especially in real-time systems where conditions are highly dynamic.
Space Complexity
Space complexity captures how the memory consumption of an algorithm grows in relation to the input size. It's another critical dimension that merits careful consideration during implementation. Efficient memory usage can dictate not just the feasibility of an algorithm but also its speed and overall performance. A program that optimally manages its memory resources can execute significantly faster than others that do not prioritize this aspect. A balance struck between space and time complexity is paramount for effective algorithm design.
Real-World Applications
In the realm of computer science, the application of data structures often determines the efficiency and effectiveness of various systems and processes. As we explore real-world applications, it becomes clear that these structures are not mere academic conceptsâthey are vital to the functioning of everyday software that individuals and organizations rely on.
Data Management Systems
Data management systems are essential for organizing, storing, and retrieving data in an efficient manner. In these systems, the choice of data structure greatly influences performance and scalability. For instance, databases commonly utilize B-trees and hash tables to enable fast lookup and retrieval operations. By structuring data effectively, engineers can ensure that even massive datasets are handled smoothly, allowing for quick queries and updates.
- Benefits:
- Enhanced performance for large data sets
- Quick access and modification of data
- Facilitation of complex queries through optimized structures
Consider the impact of structured data in a library management system. By using linked lists to track borrowed items and hash tables for easy book lookup, the system can process borrowing and returning of books, seamlessly handling multiple users without bottlenecks. This structured approach becomes increasingly valuable as user numbers grow.
Network Routing Protocols
In network communications, routing protocols benefit significantly from data structures, which aid in managing routes and connections as information traverses through the internet. Most commonly, network routers use structures like graphs to represent pathways between nodes. Each node might represent a router or a destination, with edges denoting potential paths data can take.
- Considerations for implementation:
- The choice of data structures affects latency and speed of data transfer
- Graph algorithms can provide optimal pathfinding solutions, such as Dijkstra's algorithm
For example, in an ISP's infrastructure, using graphs allows for the visualization of network topology. This means they can quickly determine the best route for data packets. An advanced implementation could involve dynamic updating of these structures to reflect real-time changes in the network, ensuring constant optimization.
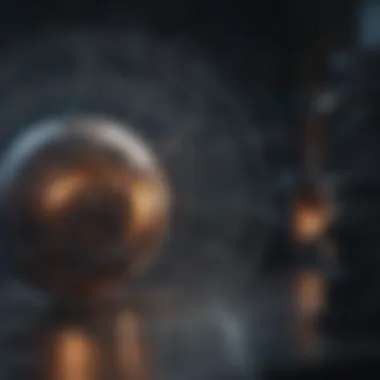
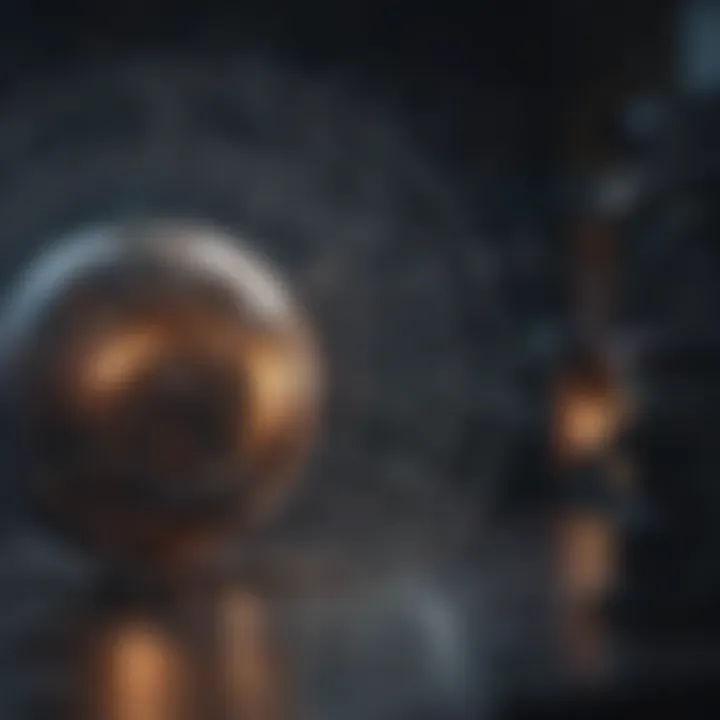
Artificial Intelligence
Artificial Intelligence is another area where data structures play a pivotal role. Here, the organization and processing of vast datasets are crucial for machine learning algorithms and neural networks. Structures like trees or heaps can be utilized to manage decision-making processes and data categorization effectively. The efficiency of algorithms in AI is deeply influenced by the data structure choices made during implementation.
- Why it matters:
- Affects the performance of machine learning models
- Enables organized processing of large volumes of data
- Facilitates faster learning and adaptation of AI systems
When building AI models, a well-structured dataset allows for more efficient training processes. For instance, using matrices for image recognition tasks can improve both speed and accuracy, enabling models to discern patterns in data without getting bogged down.
"The choice of data structure is not just an implementation detail; it's a fundamental decision that impacts the entire architecture of software systems across industries."
As we examine these real-world applications, it's evident that the implementation and choice of data structures are not simply academic exercises. They are fundamental components that impact the performance and capabilities of systems across the board, from managing simple databases to complex AI systems.
Challenges in Implementation
Understanding the challenges in the implementation of data structures is paramount for anyone navigating the labyrinth of computer science. These challenges donât just appear out of thin air; they are intertwined with both the technology we use and the design choices we make. When developing software, there's a constant tug-of-war between efficiency, performance, and the complexities that arise during implementation. Each decision can ripple through the entire system, impacting memory usage, speed, and the eventual scalability of applications. Therefore, addressing these challenges head-on is essential for delivering robust solutions.
Memory Management Issues
Memory management issues persist as one of the trickiest aspects when dealing with data structures. In programming, allocating and deallocating memory efficiently is crucial. Imagine a scenario where youâre running a program that relies heavily on linked lists. If the memory isn't managed right, it can lead to significant leaks, which would be as effective as trying to hold water in a sieve!
Memory leaks occur when a program fails to release memory that is no longer needed, which could slow down the system or even crash it altogether. If you have ever encountered a sluggish application, you might be witnessing the aftermath of poor memory allocation.
In languages like C++, manual memory management puts the onus on developers to ensure that every bit is accounted for. This meticulousness can lead to potential pitfalls down the road, presenting risks that may not surface until the application is under fire.
Here are some common strategies to tackle memory management issues:
- Garbage Collection: Automatically reclaims memory, abstracting the complexity from developers.
- Memory Pools: Allocates fixed-size blocks, reducing fragmentation.
- Smart Pointers (in C++): Helps manage the lifetime of objects, ensuring memory is freed appropriately.
The key takeaway is that understanding the memory footprint of your data structures can prevent costly errors and performance degradation.
Scalability Concerns
Scalability concerns arise when data structures begin to buckle under pressure, particularly as the volume of data swells. When a system is designed without foresight into how it will handle increased loads, itâs akin to building a house without considering the seasonsâit may withstand the mild weather, but flounder in a storm.
Choosing the right data structure from the get-go is critical. For instance, a naive array might serve well for certain operations but can become a bottleneck when the data size expands. In contrast, opting for a hash table may facilitate quicker lookups, but it's not without it's fluctuations in performance with larger datasets.
When addressing scalability, developers should consider the following:
- Data Partitioning: Distributing data across multiple nodes to balance load.
- Choice of Data Structure: Considering a trade-off between speed and capacity is essential.
- Monitoring Performance: Employing tools to continually assess how data structures behave as data evolves.
"Choosing the right data structure is akin to setting the foundation of a house; it can make or break the stability of your entire system."
Both memory management and scalability highlight the nuanced balancing act that developers must navigate. As the landscape of data continually shifts, staying adaptive and informed on these issues can significantly ease the burden of implementation, setting the stage for innovation in data structures.
Future Trends in Data Structures
The rapidly evolving technological landscape necessitates a preemptive look at potential advancements within data structures. This section investigates emerging trends that could significantly impact the effectiveness, efficiency, and applicability of data structures across numerous fields. Understanding these trends allows developers, educators, and researchers to stay ahead of the curve and anticipate changes that may redefine the frameworks we currently use.
Quantum Data Structures
As quantum computing continues to gain momentum, the notion of quantum data structures is becoming increasingly pertinent. Unlike classical data structures that operate within classical computing frameworks, quantum data structures take advantage of the peculiar properties of quantum mechanics, such as superposition and entanglement. This means that data can be represented and manipulated in ways previously thought impossible.
A key aspect of quantum data structures lies in their potential to dramatically enhance certain functional operations. For instance, quantum search algorithms such as Groverâs Algorithm offer profound speed advantages over classical search methods. With the correct quantum data structure, one could traverse vast datasets in a fraction of the time it typically would take.
However, these structures also come with unique challenges. The very nature of quantum states makes them delicate and susceptible to errors, leading to the necessity of sophisticated error correction methods. Moreover, as researchers begin to develop usable quantum data structures, practical implementations in real-world applications remain largely theoretical at this stage. Current efforts focus on understanding how to effectively blend classical and quantum paradigms, ensuring compatibility as quantum technologies mature.
Artificial Intelligence Enhancements
Artificial intelligence (AI) is not just about crafting intelligent algorithms; itâs also deepening how we understand and utilize data structures. AI can refine data structuring through machine learning models, optimizing how data is organized and accessed, driving efficiency. For example, self-organizing data structures can adapt based on the access patterns users establish over time, leading to predictions about how data should be organized in the future.
Additionally, AI enhances search capabilities. By integrating natural language processing, data structures can be reshaped to support more intuitive interactions. Users could pose questions in everyday language, and AI can dissect data structures to retrieve precise answers. This marks a departure from rigid querying methods, paving the way for more user-friendly interactions.
It's vital to consider that these enhancements don't come without a cost. The intertwining of AI with data structures introduces concerns about data privacy and ethical handling of information. As we move forward, designers and programmers must weave ethical considerations into their data manipulation strategies, ensuring that while they enhance efficiency and accessibility, they do not compromise user trust.
In the end, both quantum and AI-enhanced data structures stand at the cutting edge of research and application, challenging our traditional understanding and encouraging innovative approaches to information management.
Ending
In the realm of computer science, understanding the concrete role of data structures is pivotal. This concluding section ties together the vast array of concepts discussed throughout the article. The meticulous analysis of various data structures and their applications amplifies how they contribute fundamentally to the efficiency of algorithms and overall system performance.
Recap of Key Points
To summarize the essential takeaways from this discussion:
- Defining Data Structures: We started by establishing what data structures are, highlighting their core function in organizing and managing data effectively.
- Types of Data Structures: The classification into linear and non-linear structures provided a comprehensive overview, clarifying scenarios in which each type excels.
- Fundamental Operations and Implementation Strategies: We navigated through critical operations such as insertion, deletion, and traversal techniques, linking them to practical programming languages like C++, Python, and Java. Each language employs unique approaches yet shares the same foundational principles.
- Performance Analysis: Understanding time and space complexity is crucial in assessing the efficiency of chosen data structures in handling various computational tasks. Recognizing how performance impacts real-world applications in areas such as artificial intelligence and data management systems cannot be overstated.
- Challenges in Implementation: Addressed the pressing concerns of memory management and scalability in todayâs ever-evolving tech landscape.
- Future Trends: Speculations on quantum data structures and enhancements through artificial intelligence offer intriguing directions for future exploration.
- Linear structures, like arrays and stacks, offer straightforward relationships between elements.
- Conversely, non-linear structures like trees and graphs cater to more complex data relationships.
Implications for Future Research
Future studies should delve deeper into integrating emerging technologies with traditional data structures. Significant areas for further research include:
- The adaptation of data structures to better fit the needs of big data analytics, where scale and speed are paramount.
- Exploration of hybrid models that combine the strengths of different data structures, potentially yielding efficient, robust solutions for contemporary challenges.
- Analysis of how machine learning algorithms could refine the selection of data structures algorithmically, optimizing efficiency based on specific data behavior and structure types.
"Embracing new paradigms in data structures can pave the way for breakthroughs in how we approach complex system design and problem-solving."